IDC2 - Dystopian Mecha Carnage Ballet
Summary
For IDC2 I chose to evolve my IDC1 idea into a new and improved form instead of creating something completely new, as I knew I could push the boundaries
of my capabilities more. The initial problem I set out to solve still remained, my room's temperature is terribly regulated; the heating is inefficient, there's no AC for the summers, and
I prefer to sleep in a very cold room so i tend to open my window at night. I realized that If I could find a way to properly automate the opening and closing of my window using arduino, I
would be able to optimize the space automatically.
My first idea was to use a system of cables and pulleys attached to spools and DC motors. This idea would be
minimalist, and has the potential to make opening the window trivial with mechanical advantage. The main issue with this idea is mounting stuff to my window,
which would require drilling, and attaching, and kissing my security deposit goodbye.
Eventually I settled on the lead screw idea. These rotational-to-linear
steppers are powerful and inexpensive, with a high degree of control. I secured one via amazon along with an A4988 stepper motor driver, a waterproof temperature sensor and 12v DC power supply.
Process
I started by mentally solving the spatial logistics by sketching in Paint. I measured my window's dimensions to visualize the width, and the relative locations of the part of the window that slides (where the attachment point lies when opened and closed). I also took the time to clean and lubricate the window's tracks to ensure the stepper would not struggle to operate
Next up was to construct the bulk of the machine. I chose wood as my material of choice because it provides the necessary weight and rigidity to handle high strain while being very easy to work with. Using ITLL scrap wood and tools I attached the stepper motor mount with two screws through the bottom. Fearing the possibility of structural failure, I also glued in two triangular struts to ensure the mount was strong and stiff. I let the glue dry completely before continuing
When the glue had hardened I began wiring the electronics. Using the internet as my guide I carefully wired the stepper motor through my A4988. This required many hours of tedious fine tuning. The driver has a small screw (potentiometer) on the top face that can be turned to adjust the amperage. For my specific stepper motor, this needed to be tuned to 0.8 amps to optimize motor power while avoiding overheating. When I was able to drive the motor, I phased in my temperature sensor and fans (the same 5v USB set from IDC1 to save money).
Finally, the code. Starting from some example code I found on the internet, I built up my program. Establishing my pins, calling my libraries, and fine tuning my delays, I achieved my first
successful test. The most challenging part of this was a simple bug that would haunt me later. When the arduino first establishes a connection with the temperature sensor,
the first number that appears in the serial monitor is always 185°F. I belive this is a bug within the library that controls the sensor, but essentially, any reset in the arduino
immediately blows through the temperature threshold and the machine reacts by opening the window (as it was designed to do). Unfortunately, if the adapter that attaches to the
window is already in the opened state, it is pulled in and collides with the stepper.
Once my electronics were complete I manufactured a cover out of wood with holes for the incoming and outgoing wires, and placed it on.
Then it was time to present my build on the fateful Friday morning. Who cares if it's reading day, my academic prowess will be put to the test regardless.
Midway through my demonstration, once the machine had "opened" the window, I noticed the fans were not turning on. I reached down and flipped the fans into high. Unfortunately,
this sudden pull of voltage, likely due to the strained arduino which was powering three things despite having two power output pins, reset the arduino.
Like a farmer watching my favorite sheep get devoured by a combine, the sudden spike in perceived temperature caused the machine to open the window again. The subsequent
disaster was described verbally by the very disappointed professor Weaver as akin to "mechanical performance art where the machine breaks itself". I did not
stay around to watch the second round of presentations.
In order to get around this bug, I hard coded in a method to avoid double openings/closings, as
well as a system that requires three consecutive temperature readouts above or below the threshold in order to begin the opening/closing process.
Images
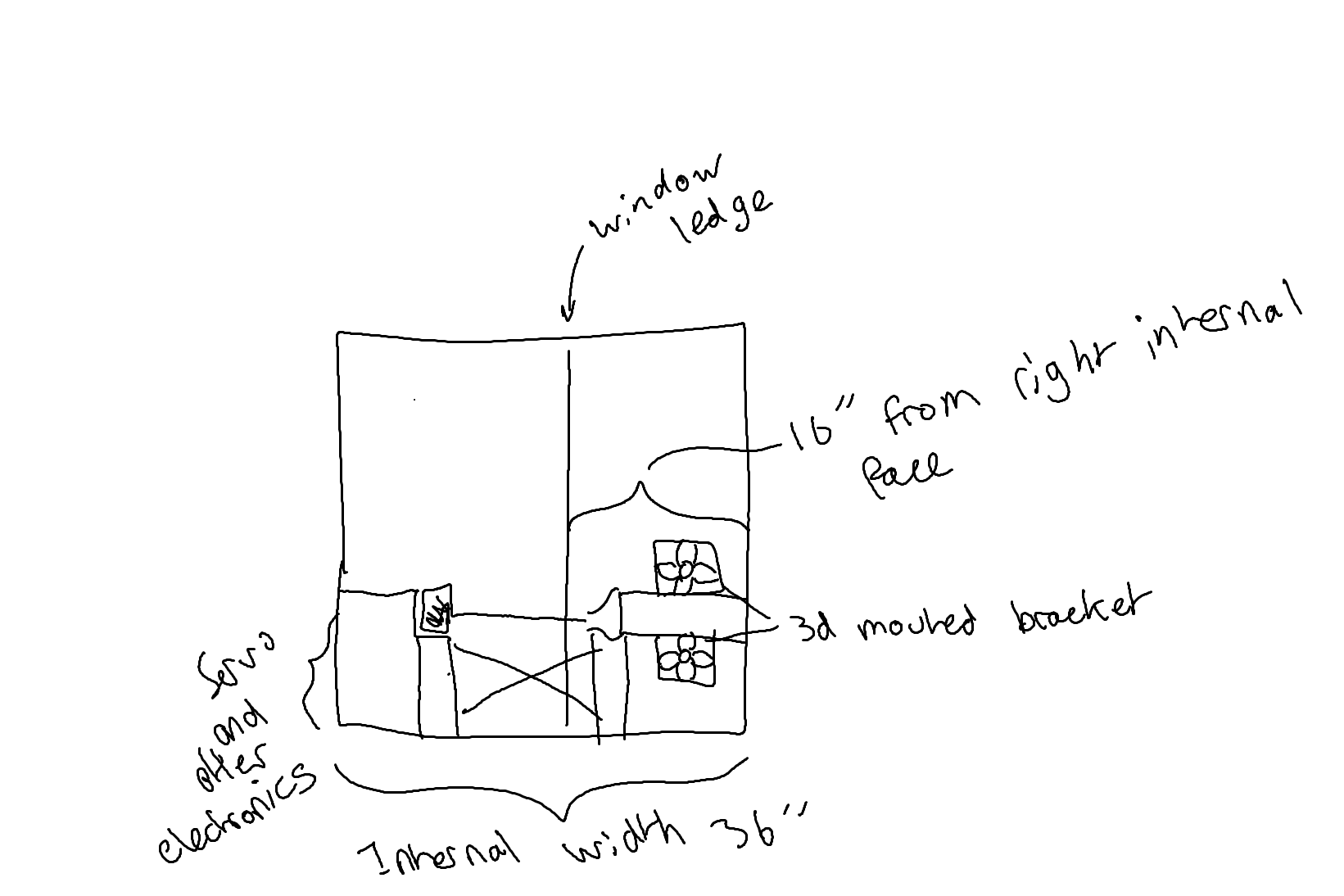
Initial sketch in Paint
Finished wooden frame // First motor tests
Wiring the stepper
Finished wooden frame // First motor tests.
Wiring the A4988 motor driver
Wiring the A4988 motor driver CONTD
Videos
First successful test
Working machine installed in bedroom window
Code
#include
#include
//pins
#define ONE_WIRE_BUS 4 // data wire for temperature sensor
#define stepPin 2 // step pin for stepper motor
#define dirPin 5 // direction pin for stepper motor
//thresholds
const float UPPER_THRESHOLD = 80.0; // farenheit
const float LOWER_THRESHOLD = 77.0; // Farenheit
// temp sensor library
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
//track window state
bool windowOpen = false; // 0 = Closed, 1 = Open
// Consecutive counter
int aboveThresholdCount = 0;
int belowThresholdCount = 0;
const int REQUIRED_CONSECUTIVE_READINGS = 3;
// driving the stepper motor
void rotateMotor(bool direction, unsigned long duration) {
digitalWrite(dirPin, direction ? HIGH : LOW);
unsigned long startTime = millis();
while (millis() - startTime < duration) {
digitalWrite(stepPin, HIGH);
delayMicroseconds(700); // step timing
digitalWrite(stepPin, LOW);
delayMicroseconds(700);
}
}
void setup() {
Serial.begin(9600);
while (!Serial) {
; // wait for serial port
}
sensors.begin();
pinMode(stepPin, OUTPUT);
pinMode(dirPin, OUTPUT);
Serial.println("Initializing Temperature Sensor...");
int deviceCount = sensors.getDeviceCount();
Serial.print("Found ");
Serial.print(deviceCount);
Serial.println(" temperature sensor(s)");
}
void loop() {
float tempF;
sensors.requestTemperatures();
tempF = sensors.getTempFByIndex(0);
if (tempF == DEVICE_DISCONNECTED_F) {
Serial.println("Error: Temperature sensor disconnected!");
delay(1000);
return;
}
Serial.print("Current Temperature: ");
Serial.print(tempF, 2);
Serial.println(" °F");
// logic for opening and closing the window with consecutive checks
if (tempF > UPPER_THRESHOLD) {
aboveThresholdCount++;
belowThresholdCount = 0; // Reset below count
if (aboveThresholdCount >= REQUIRED_CONSECUTIVE_READINGS && !windowOpen) {
Serial.println("Opening window");
rotateMotor(true, 5000); // drive motor to open window
windowOpen = true;
aboveThresholdCount = 0; // reset counter IMPORTANT
}
}
else if (tempF < LOWER_THRESHOLD) {
belowThresholdCount++;
aboveThresholdCount = 0; // Reset above count
if (belowThresholdCount >= REQUIRED_CONSECUTIVE_READINGS && windowOpen) {
Serial.println("Closing window");
rotateMotor(false, 5000); //drive motor to close window
windowOpen = false;
belowThresholdCount = 0; // reset counter IMPORTANT
}
}
else {
aboveThresholdCount = 0;
belowThresholdCount = 0;
}
delay(1000); // Delay between temperature checks (serial monitor)
}