Design 1: Honeycomb
Processing Code
import Turtle.*;
import processing.svg.*;
Turtle t;
boolean recording = false;
void setup() {
size(700, 700);
noLoop(); // Prevent continuous drawing
drawPattern();
}
void drawPattern() {
background(255);
t = new Turtle(this);
t.setHeading(30);
float l = 25; // Side length
int cols = (int) ceil(width / (3 * l));
int rows = (int) ceil(height / (sqrt(3) * l * 0.5));
for (int row = 0; row < rows; row++) {
for (int col = 0; col < cols; col++) {
t.penUp();
float x = col * (3 * l) + (row % 2) * (1.5 * l);
float y = row * (sqrt(3) * l * 0.5);
if (x < width && y < height) {
t.goToPoint(x, y);
t.penDown();
float randomL = random(-8, 8);
for (int side = 0; side < 6; side++) {
t.forward(l - randomL);
t.right(60);
}
}
}
}
}
void keyPressed() {
if (key == 's') {
String fileName = "output/honeycomb-" + getDateString() + ".svg";
beginRecord(SVG, fileName);
drawPattern();
endRecord();
println("Saved to file: " + fileName);
}
}
String getDateString() {
return year() + "_" + month() + "_" + day() + "-" + hour() + "_" + minute() + "_" + second();
}
Images
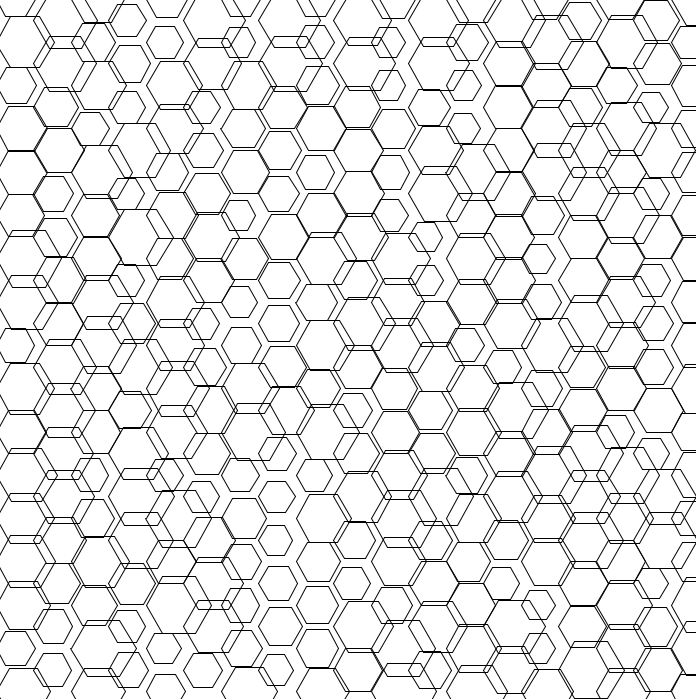
Rendered output of Design 1 in Processing. The size of a given hexagon is randomized
This code mathematically constructs a honeycomb grid by calculating the necessary number of columns and rows based on the canvas
dimensions and hexagon side length. The spacing follows a structured logic: each hexagon’s center is positioned using a formula
that ensures proper staggered alignment. Horizontally, hexagons are placed every 3l units, ensuring they interlock correctly.
Vertically, they are spaced by frac{sqrt{3}}/{2} times l , preserving the honeycomb pattern. Additionally, every alternate
row shifts hexagons right by 1.5l to maintain the staggered structure. In addition, a slight random variation is introduced to side lengths,
adding organic imperfections to each hexagon for the visual effect.